Mobile developers can use Apple Wallet and Google Wallet integrations for different types of apps:
-
Boarding pass storage and hotel reservations;
-
Card for short-term rental;
-
Passes for buildings or events;
-
Scan tickets for travel or subscriptions;
-
Store loyalty cards and gift vouchers;
-
Providing contactless payment, etc.
Once we already wrote our own library for Apple Wallet in React Native because we couldn’t find anything ready to use. But now that Google Wallet is fully released, it’s time to update it.
Now, this library provides integration with both Apple Wallet on iOS and Google Wallet on Android. It allows you to add, remove, and check for existing passes on iOS, and add passes to Google Wallet on Android.
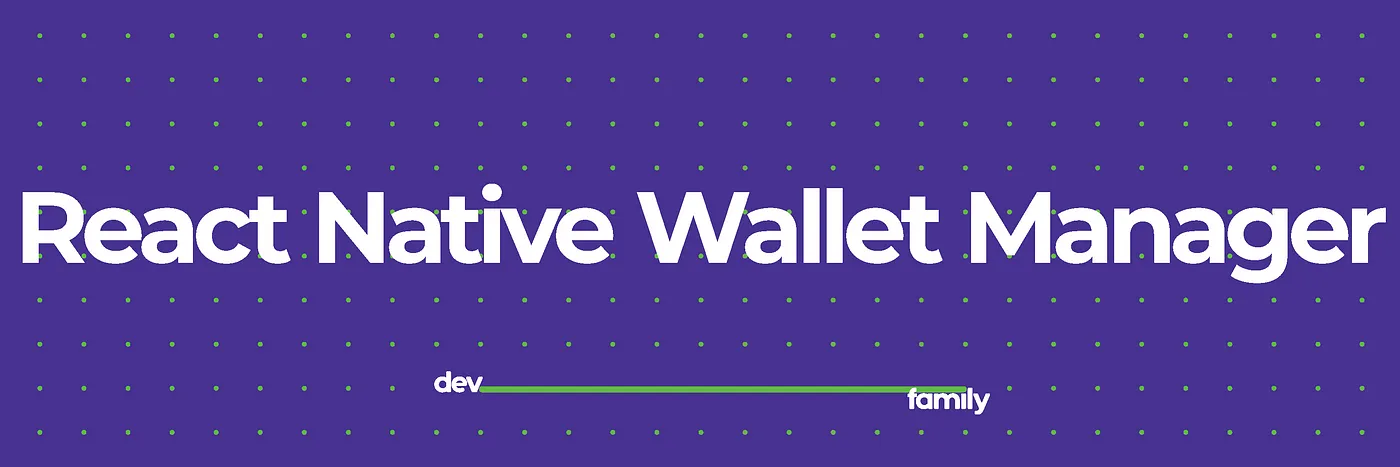
react-native-wallet-manager
This library provides integration with both Apple Wallet on iOS and Google Wallet on Android. It allows you to add, remove, and check for existing passes on iOS, and add passes to Google Wallet on Android.

iOS

Android
Installation
yarn add react-native-wallet-manager
or
npm install react-native-wallet-manager
Setup
iOS
To enable the Wallet functionality on iOS, you need to add the Wallet capability in Xcode. Follow these steps:
-
Open your project in Xcode (
xcworkspace
file). -
Select your project Target.
-
Go to the Signing & Capabilities tab.
-
Click on + Capability to add a new capability.
-
In the search bar, type Wallet and add it to your project.
After that, navigate to the ios
folder in your project directory and run the following command to install necessary dependencies:
cd ios && pod install
Android
To enable Google Wallet functionality on Android, you need to add the Google Play Services dependency to your project. Follow these steps:
-
Open the
android/app/build.gradle
file in your project. -
In the
dependencies
section, add the following line with the latest version ofplay-services-pay
:implementation "com.google.android.gms:play-services-pay:<latest_version>"
Replace <latest_version>
with the most recent version number available. You can find the latest version on the Google Play Services release notes page.
Usage
For platform-specific instructions on how to create passes:
-
iOS: For a detailed guide on creating Apple Wallet pkpasses visit this Medium article.
-
Android: You can find a detailed guide on creating passes for Google Wallet at this Google Codelab.
To use this library, start by importing it into your React Native component:
import WalletManager from 'react-native-wallet-manager';
API

Notes
All methods return a Promise
that resolves with the result of the operation or rejects if an error occurs.
canAddPasses()
Checks if the device can add passes to the wallet. This method is supported on both iOS and Android.
Example
const canAddPasses = async () => { try { const result = await WalletManager.canAddPasses(); console.log(result); } catch (e) { console.log(e); } };
addPassToGoogleWallet(jwt: string)
Adds a pass to Google Wallet. This method is only supported on Android.
Parameters
-
jwt
(string): The JWT token containing the pass information to be added to Google Wallet.
Note: In the example below, you’ll see a placeholder for the JWT. You need to generate a valid Google Wallet JWT for testing purposes.
Example
const addPassToGoogleWallet = async () => { try { await WalletManager.addPassToGoogleWallet( 'eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9...' ); } catch (e) { console.log(e); } };
addPassFromUrl(url: string)
Adds a pass from a specified URL. This method is supported on both iOS and Android.
-
On iOS, it will add the pass to Apple Wallet.
-
On Android, it will open the URL in a browser.
Parameters
-
url
(string): The URL of the pass file (.pkpass
file) to be added.
Examples
const addPass = async () => { try { const result = await WalletManager.addPassFromUrl( 'https://github.com/dev-family/react-native-wallet-manager/blob/main/example/resources/pass.pkpass?raw=true' ); console.log(result); } catch (e) { console.log(e); } };
hasPass(cardIdentifier: string, serialNumber?: string)
Checks if a pass with a specific identifier exists in the Apple Wallet. This method is only supported on iOS.
Parameters
-
cardIdentifier
(string): The unique identifier of the pass to check. -
serialNumber
(string, optional): The serial number of the pass. This parameter is optional and can be used to further specify the pass.
Example
const hasPass = async () => { try { const result = await WalletManager.hasPass('pass.family.dev.walletManager'); console.log(`has pass: ${result}`); } catch (e) { console.log(e, 'hasPass'); } };
removePass(cardIdentifier: string, serialNumber?: string)
Removes a pass with a specific identifier from the Apple Wallet. This method is only supported on iOS.
Parameters
-
cardIdentifier
(string): The unique identifier of the pass to remove. -
serialNumber
(string, optional): The serial number of the pass. This parameter is optional and can be used to further specify the pass.
Example
const removePass = async () => { try { const result = await WalletManager.removePass( 'pass.family.dev.walletManager' ); console.log(`remove pass: ${result}`); } catch (e) { console.log(e, 'removePass'); } };
viewInWallet(cardIdentifier: string, serialNumber?: string)
Opens a pass with a specific identifier in Apple Wallet. This method is only supported on iOS.
Parameters
-
cardIdentifier
(string): The unique identifier of the pass to view. -
serialNumber
(string, optional): The serial number of the pass. This parameter is optional and can be used to further specify the pass.
Example
const viewPass = async () => { try { const result = await WalletManager.viewInWallet( 'pass.family.dev.walletManager' ); console.log(result); } catch (e) { console.log(e, 'error Viewing Pass'); } };
showViewControllerWithData(filePath: string)
Displays a view controller to add a pass using the data from the specified file path. This method is only supported on iOS.
Parameters
-
filePath
(string): The path to the.pkpass
file containing the pass data to be added to Apple Wallet.
Example
const showViewControllerWithData = async (filePath) => { try { const result = await WalletManager.showViewControllerWithData(filePath); console.log(`Pass was added: ${result}`); } catch (e) { console.error('Error displaying pass view controller:', e); } };
Project inspired by react-native-wallet
Contributing
See the contributing guide to learn how to contribute to the repository and the development workflow.
License
MIT
To download the library go here.
ссылка на оригинал статьи https://habr.com/ru/articles/858858/
Добавить комментарий